VoxEngine concepts
Voximplant has a JS-based core called VoxEngine which processes applications based on JS scenarios.
VoxEngine uses the ECMAScript 6 standard JavaScript and based on the SpiderMonkey engine, that is also used in the Firefox 81 web browser. Hence, it supports all the features that this engine supports.
We recommend sticking to the ECMAScript 6 standard when writing your scenarios, and if you want to use some of the newer features, check if this feature is compatible with Firefox 81 via the Can I use website.
You can use our Cloud IDE for working with VoxEngine scenarios, or you can use your 3rd-party IDE solution. Working with 3rd-party IDEs has its advantages and disadvantages. For example, you can create scenarios with the help of technologies such as TypeScript and Webpack. However, these instruments can be configured incorrectly, resulting in not human-readable code, which makes it impossible for developers to work with their code, and for the Voximplant support team to troubleshoot and debug your applications.
This is why we recommend using VoxEngine CI when developing your scenario in a 3rd-party IDE.
Event-based model
Our scenarios use the event-based approach, which means that developers can easily manage their application logic by subscribing to events and processing them.
Please notice, that some events are nested, which means, to subscribe to them, you first need to subscribe to the parent event. For example, to process the CallEvents, such as Connected or ToneReceived, first you need to subscribe to the parent CallAlerting event, which processes calls. After you subscribe to the CallAlerting event, you receive the Call object, which has its own events.
VoxEngine.addEventListener(AppEvents.CallAlerting, (e) => {
// you receive the incoming call object in the e.call property
// the call has its own events, such as "ToneReceived"
e.call.handleTones(true); // enable processing tone signals
e.call.answer(); // answer the incoming call
//subscribe to the call's event
e.call.addEventListener(CallEvents.ToneReceived, (e) => {
e.call.say("You pushed the ${e.tone} button", {language: VoiceList.Amazon.en_US_Joanna});
});
e.call.addEventListener(CallEvents.Disconnected, () => VoxEngine.terminate());
});
Without subscribing to the parent event, the incoming call object and its events are not available. For outgoing calls from the scenario, you create the call object with its events via the call methods, for example:
VoxEngine.addEventListener(AppEvents.Started, () => {
// create a new call when scenario starts
const call = VoxEngine.callPSTN("numberToCall", "confirmedCallerId");
// get the events from the newly created object
call.addEventListener(CallEvents.Connected, () => {
call.say("Wake up, Neo!", { language: VoiceList.Amazon.en_US_Joanna });
});
});
Please note, that if you are making a call via web or mobile SDK, it consists of two call legs: SDK → scenario and scenario → destination. In this case, the SDK → scenario call leg is considered an incoming call in the scenario, so you need to process it via the CallAlerting event as well. Read the How to process calls in a scenario article to learn more about processing incoming and outgoing calls in scenarios.
Some events are consequent, which means that one event can only happen after a certain event has been triggered. For example, the media player's PlaybackFinished event, which indicates that media player has finished playing media to the call, can only happen after the player's Started event, which indicates that the media player has started playing media to the call.
Session lifecycle
Every attempt to start a call using Voximplant creates a new independent call session in VoxEngine; except for conferences, in this case, the call goes into an existing session.
A session always starts with the Started event due to an incoming call or an HTTP request.
The next event depends on what started a session: it can be the CallAlerting event in case of a call or the HttpRequest event in case of an HTTP request.
The CallEvents can be triggered during a session: when a call is connected or when it is put on hold, when call recording is started, and so on.
Normally, the session’s ending is executed in the next steps:
- The terminate method is called, an error occurs, or a session ends after timeout if there are no active calls and ACD requests.
- The Terminating event is triggered. Timers and any other external resources are not available after this event is triggered, but you can perform one HTTP request inside the event handler (e.g., to notify an external system about the fact that the session is finished). When that request is finished.
- The Terminated event is triggered.
A session without calls and ACD requests is terminated after 60 seconds.
To learn about session limits, check out the Limits and restrictions article.
Standard library
VoxEngine supports basic functions such as setTimeout, clearTimeout, str2Bytes, base64_encode, uuidgen, a set of crypto methods, etc. But they can be slightly different from their originals even in the names. Please look them up in the VoxEngine reference before using them to make your scenario work properly.
Logging
Use the Logger.write method to write messages to the session logger.
When a session terminates, its log immediately becomes available in Call history. To access it, you can either go to the Calls section in the Control panel or use the GetCallHistory API method. Retrieving logs via the Control panel, you get access to a handful of visual representation:
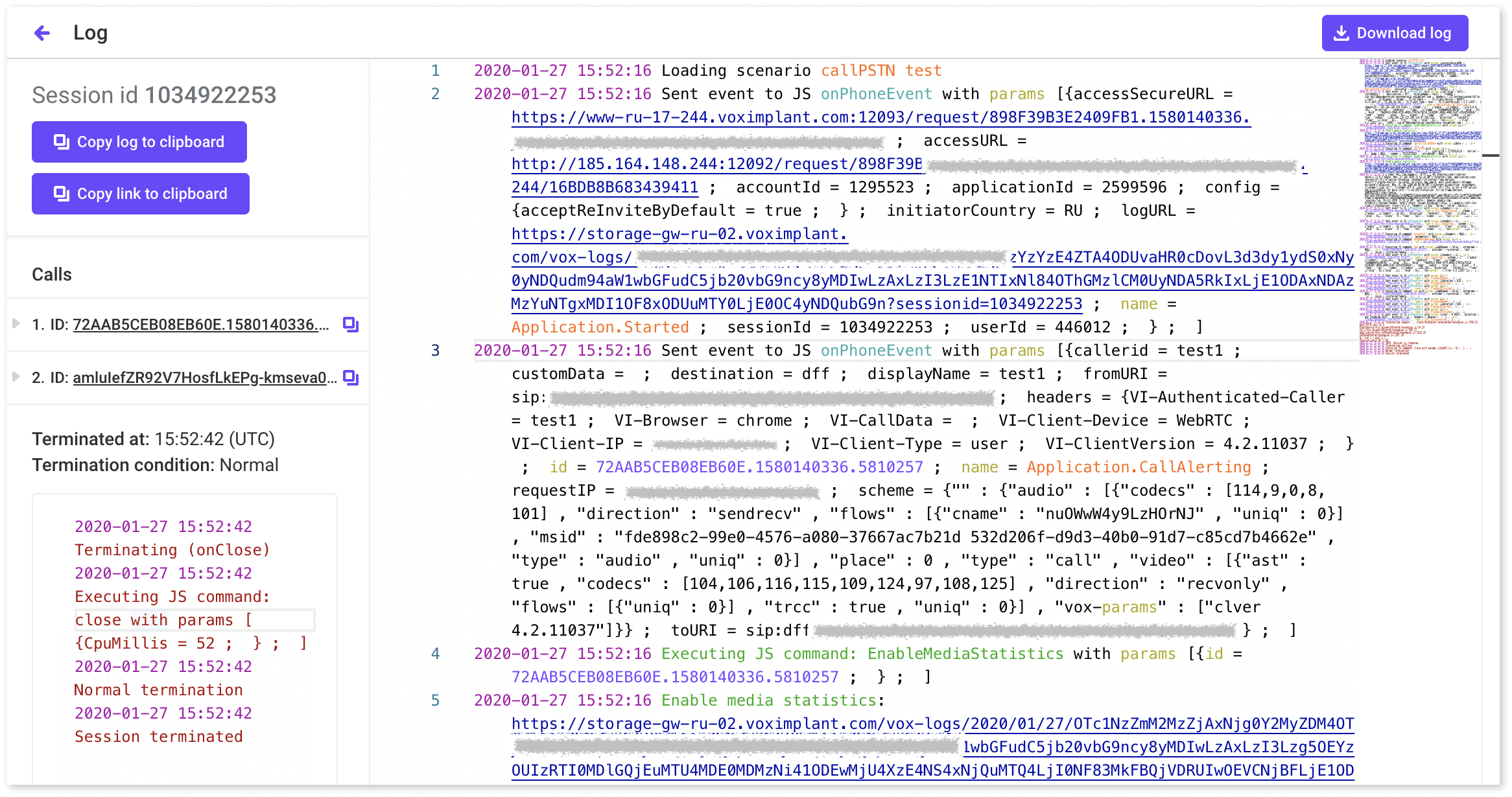
Key features
Copying a log itself or the link to it to the clipboard
Downloading logs in the .txt format – see the button in the top right corner
Events and their time codes are highlighted for readability
Navigating through long logs with the help of a minimap in the top right corner
Hiding any of a call's sides (legs) for more convenient analysis – unfold the needed leg on the left side of the screen and uncheck the Highlight call lines box there.
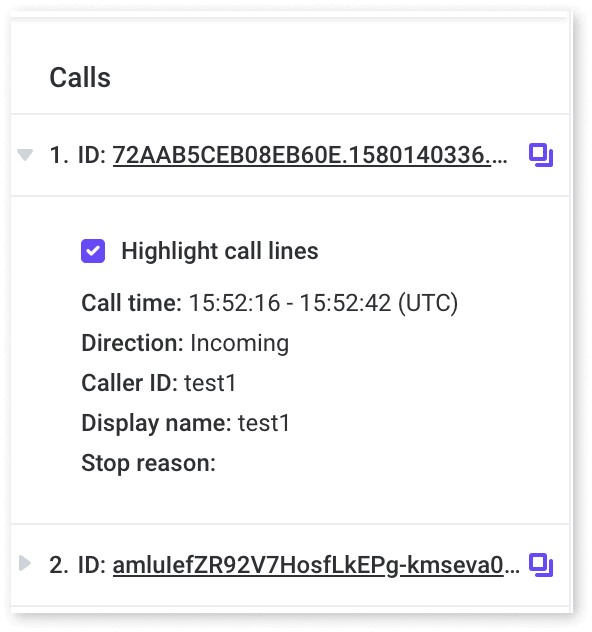